Concurrency vs. parallelism: How Python’s asyncio makes the difference?
- Shaikh N
- Sep 30, 2024
- 3 min read
In Python, especially in the world of async programming tasks terms such as concurrency and parallelism are very commonly used. What do these say though? How does Python's `asyncio` module fit into this question — more on that below. Breaking it down in simple terms.
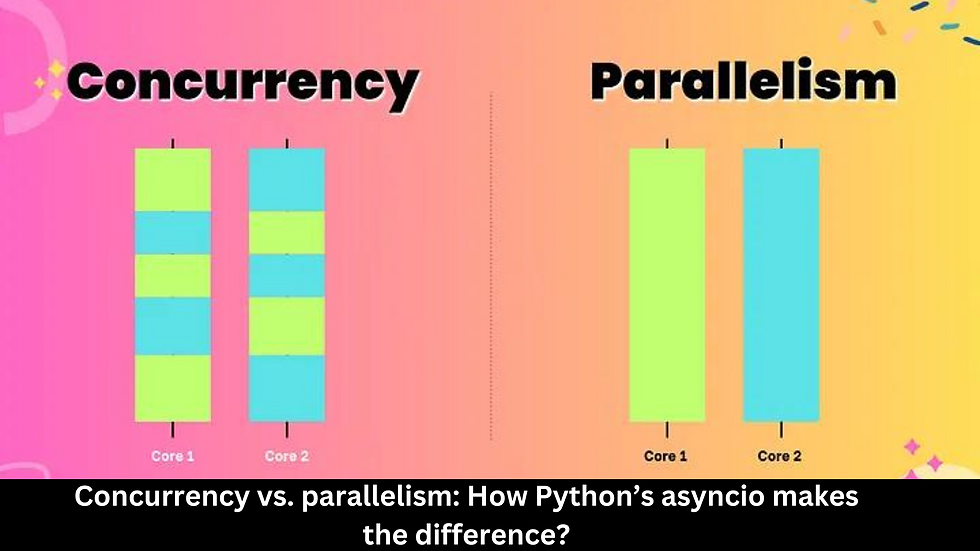
Concurrency and parallelism, not quite the same thing
Although similar, there is a distinction between concurrency and parallelism. Concurrency is multitasking. This work is not going on all at the same time but instead, push around to allow the system to switch between them as needed in an organised fashion. Think of it like you're texting a friend while refreshing your email for an important mail. You are doing one thing at a time, but back and forth between the two. That’s concurrency.
Parallelism, on the other hand, is when multiple independent tasks are actually happening simultaneously. This generally means different cores of a CPU. It’s like having 2 people in the kitchen 1st chopping vegetables and 2nd boiling Pasta both actions happen at once.
In short, concurrency is very task driven and parallelism is more so execution. Concurrency is what is used to deal with multiple processes, and parallelism means conducting them all together.
Concurrency with Python: `asyncio`
Python is famous for being an accessible and beginner language, however, handling async code can be tough. That is where asyncio becomes relevant.
asyncio is a Python module that provides events, coroutines and network functionality without the use of threads or blocking. It gives you the possibility to sort out parallel duties with no obstruction in performing them at a time. This is beneficial when you are working around I/O-bound operations such as pulling data from a server or reading files on disk. Instead of waiting for one task to finish before starting another, you can use `asyncio` to handle multiple tasks at once, making your program more efficient.
Note that `asyncio` helps you manage tasks concurrently, but it won't mean they are running in parallel. The `asyncio` library in Python uses an event loop to make sure, for example, that one task can wait while another keeps running. This enables your code to be non-blocking and very responsive, while actually running multiple tasks at the same time.
Asyncio and concurrency: A perfect match
Alright then, where does `asyncio` stand in this model of concurrency? It is the ability to perform adequately in a range of tasks, without expending much time on the overhead associated with using threads or processes. Whereas in multi-threading multiple threads are used to carry out tasks, `asyncio` works with coroutines that wait until a desirable state is reached.
Let's take an example, you have a web scraping application that has to fetch some data from multiple sites. With synchronous programming, you would have to wait for a response from each website one at a time which can be forever. But with asyncio we can make all requests at the beginning and start collecting their results as soon as data becomes available.
On the other hand, `asyncio` is perfect for I/O-bound tasks like network requests or reading from / writing to files. Moreover, it leaves your code simple to manage without the complexities and potential bugs of multi-threading.
When should you use parallelism in place of concurrency?
That said, `asyncio` is perfect for concurrency but not at all suitable for CPU-bound tasks. If your program has to do a lot of heavy calculations, then maybe concurrency is not enough. For authentic parallelism, you should research multi-processing that allows to run multiple processes on different CPU cores simultaneously.
Conclusion
However, learning the difference between concurrency and parallelism will allow you to program Python better. Concurrency is more about managing multiple tasks, and parallelism is running those tasks simultaneously. For common I/O-bound operations, Python has the `asyncio`, which makes it easy to write non-blocking code by abstracting away everything you need. If you find yourself needing additional expertise, you can hire offshore Python developers to assist with these tasks. Therefore, whether you work with network requests programming or file manipulations, understanding when to parallelize (concurrency) the code vs. using parallelism can give an enhancement in your application execution.
Comments